mirror of
https://github.com/signalapp/libsignal.git
synced 2024-09-20 03:52:17 +02:00
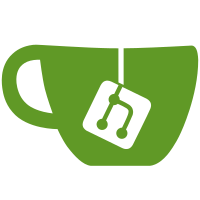
Expose message backup at the bridge layer as a separate async function. Add a TS wrapper with the same interface as for the other app languages.
94 lines
2.4 KiB
TypeScript
94 lines
2.4 KiB
TypeScript
//
|
|
// Copyright 2024 Signal Messenger, LLC.
|
|
// SPDX-License-Identifier: AGPL-3.0-only
|
|
//
|
|
|
|
/**
|
|
* Message backup validation routines.
|
|
*
|
|
* @module MessageBackup
|
|
*/
|
|
|
|
import * as Native from '../Native';
|
|
import { Aci } from './Address';
|
|
import { InputStream } from './io';
|
|
import { bufferFromBigUInt64BE } from './zkgroup/internal/BigIntUtil';
|
|
|
|
export type InputStreamFactory = () => InputStream;
|
|
|
|
/**
|
|
* Result of validating a message backup bundle.
|
|
*/
|
|
export class ValidationOutcome {
|
|
/**
|
|
* A developer-facing message about the error encountered during validation,
|
|
* if any.
|
|
*/
|
|
public errorMessage: string | null;
|
|
|
|
/**
|
|
* Information about unknown fields encountered during validation.
|
|
*/
|
|
public unknownFieldMessages: string[];
|
|
|
|
/**
|
|
* `true` if the backup is valid, `false` otherwise.
|
|
*
|
|
* If this is `true`, there might still be messages about unknown fields.
|
|
*/
|
|
public get ok(): boolean {
|
|
return this.errorMessage == null;
|
|
}
|
|
|
|
constructor(outcome: Native.MessageBackupValidationOutcome) {
|
|
const { errorMessage, unknownFieldMessages } = outcome;
|
|
this.errorMessage = errorMessage;
|
|
this.unknownFieldMessages = unknownFieldMessages;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Key used to encrypt and decrypt a message backup bundle.
|
|
*/
|
|
export class MessageBackupKey {
|
|
readonly _nativeHandle: Native.MessageBackupKey;
|
|
|
|
/**
|
|
* Create a public key from the given master key and ACI.
|
|
*
|
|
* `masterKeyBytes` should contain exactly 32 bytes.
|
|
*/
|
|
public constructor(masterKeyBytes: Buffer, aci: Aci) {
|
|
this._nativeHandle = Native.MessageBackupKey_New(
|
|
masterKeyBytes,
|
|
aci.getServiceIdFixedWidthBinary()
|
|
);
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Validate a backup file
|
|
*
|
|
* @param backupKey The key to use to decrypt the backup contents.
|
|
* @param inputFactory A function that returns new input streams that read the backup contents.
|
|
* @param length The exact length of the input stream.
|
|
* @returns The outcome of validation, including any errors and warnings.
|
|
* @throws IoError If an IO error on the input occurs.
|
|
*/
|
|
export async function validate(
|
|
backupKey: MessageBackupKey,
|
|
inputFactory: InputStreamFactory,
|
|
length: bigint
|
|
): Promise<ValidationOutcome> {
|
|
const firstStream = inputFactory();
|
|
const secondStream = inputFactory();
|
|
return new ValidationOutcome(
|
|
await Native.MessageBackupValidator_Validate(
|
|
backupKey,
|
|
firstStream,
|
|
secondStream,
|
|
bufferFromBigUInt64BE(length)
|
|
)
|
|
);
|
|
}
|