mirror of
https://github.com/mpv-player/mpv.git
synced 2024-09-19 19:42:24 +02:00
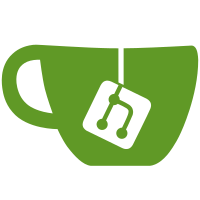
Previously, all stream protocols were a static list in mpv. This is okay for own builtin stuff, but for protocols that depend on ffmpeg it's not so great. Support for certain protocols may or may not be enabled in a user's ffmpeg and the protocol list that mpv generates should ideally match this. Fix this by implementing a get_protocols method for stream_lavf that will have different results depending on the ffmpeg mpv is built against. We keep the safe and unsafe protocols separation. The former is essentially a whitelist. Any protocol that is found in ffmpeg but is not in the safe whitelist is considered unsafe. In the stream list, ffmpeg is moved to the bottom so any possible protocols that are added in the future don't automatically take precedence over any builtin mpv ones.
57 lines
2.2 KiB
C
57 lines
2.2 KiB
C
/*
|
|
* This file is part of mpv.
|
|
*
|
|
* mpv is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2.1 of the License, or (at your option) any later version.
|
|
*
|
|
* mpv is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with mpv. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#ifndef MP_AVCOMMON_H
|
|
#define MP_AVCOMMON_H
|
|
|
|
#include <inttypes.h>
|
|
#include <stdbool.h>
|
|
|
|
#include <libavutil/avutil.h>
|
|
#include <libavutil/rational.h>
|
|
#include <libavcodec/avcodec.h>
|
|
|
|
struct mp_decoder_list;
|
|
struct demux_packet;
|
|
struct mp_codec_params;
|
|
struct AVDictionary;
|
|
struct mp_log;
|
|
|
|
enum AVMediaType mp_to_av_stream_type(int type);
|
|
AVCodecParameters *mp_codec_params_to_av(const struct mp_codec_params *c);
|
|
int mp_set_avctx_codec_headers(AVCodecContext *avctx, const struct mp_codec_params *c);
|
|
AVRational mp_get_codec_timebase(const struct mp_codec_params *c);
|
|
void mp_set_av_packet(AVPacket *dst, struct demux_packet *mpkt, AVRational *tb);
|
|
int64_t mp_pts_to_av(double mp_pts, AVRational *tb);
|
|
double mp_pts_from_av(int64_t av_pts, AVRational *tb);
|
|
void mp_set_avcodec_threads(struct mp_log *l, AVCodecContext *avctx, int threads);
|
|
void mp_add_lavc_decoders(struct mp_decoder_list *list, enum AVMediaType type);
|
|
void mp_add_lavc_encoders(struct mp_decoder_list *list);
|
|
char **mp_get_lavf_demuxers(void);
|
|
char **mp_get_lavf_protocols(void);
|
|
int mp_codec_to_av_codec_id(const char *codec);
|
|
const char *mp_codec_from_av_codec_id(int codec_id);
|
|
bool mp_codec_is_lossless(const char *codec);
|
|
void mp_set_avdict(struct AVDictionary **dict, char **kv);
|
|
void mp_avdict_print_unset(struct mp_log *log, int msgl, struct AVDictionary *d);
|
|
int mp_set_avopts(struct mp_log *log, void *avobj, char **kv);
|
|
int mp_set_avopts_pos(struct mp_log *log, void *avobj, void *posargs, char **kv);
|
|
void mp_free_av_packet(AVPacket **pkt);
|
|
void mp_codec_info_from_av(const AVCodecContext *avctx, struct mp_codec_params *c);
|
|
|
|
#endif
|