mirror of
https://github.com/mpv-player/mpv.git
synced 2024-09-20 03:52:22 +02:00
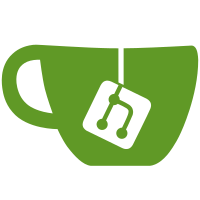
Remove them from the big MPOpts struct and move them to their sub structs. In the places where their fields are used, create a private copy of the structs, instead of accessing the semi-deprecated global option struct instance (mpv_global.opts) directly. This actually makes accessing these options finally thread-safe. They weren't even if they should have for years. (Including some potential for undefined behavior when e.g. the OSD font was changed at runtime.) This is mostly transparent. All options get moved around, but most users of the options just need to access a different struct (changing sd.opts to a different type changes a lot of uses, for example). One thing which has to be considered and could cause potential regressions is that the new option copies must be explicitly updated. sub_update_opts() takes care of this for example. Another thing is that writing to the option structs manually won't work, because the changes won't be propagated to other copies. Apparently the only affected case is the implementation of the sub-step command, which tries to change sub_delay. Handle this one explicitly (osd_changed() doesn't need to be called anymore, because changing the option triggers UPDATE_OSD, and updates the OSD as a consequence). The way the option value is propagated is rather hacky, but for now this will do.
56 lines
1.7 KiB
C
56 lines
1.7 KiB
C
#ifndef MPLAYER_SD_H
|
|
#define MPLAYER_SD_H
|
|
|
|
#include "dec_sub.h"
|
|
#include "demux/packet.h"
|
|
|
|
// up to 210 ms overlaps or gaps are removed
|
|
#define SUB_GAP_THRESHOLD 0.210
|
|
// don't change timings if durations are smaller
|
|
#define SUB_GAP_KEEP 0.4
|
|
|
|
struct sd {
|
|
struct mpv_global *global;
|
|
struct mp_log *log;
|
|
struct mp_subtitle_opts *opts;
|
|
|
|
const struct sd_functions *driver;
|
|
void *priv;
|
|
|
|
struct attachment_list *attachments;
|
|
struct mp_codec_params *codec;
|
|
|
|
// Set to false as soon as the decoder discards old subtitle events.
|
|
// (only needed if sd_functions.accept_packets_in_advance == false)
|
|
bool preload_ok;
|
|
};
|
|
|
|
struct sd_functions {
|
|
const char *name;
|
|
bool accept_packets_in_advance;
|
|
int (*init)(struct sd *sd);
|
|
void (*decode)(struct sd *sd, struct demux_packet *packet);
|
|
void (*reset)(struct sd *sd);
|
|
void (*select)(struct sd *sd, bool selected);
|
|
void (*uninit)(struct sd *sd);
|
|
|
|
bool (*accepts_packet)(struct sd *sd, double pts); // implicit default if NULL: true
|
|
int (*control)(struct sd *sd, enum sd_ctrl cmd, void *arg);
|
|
|
|
void (*get_bitmaps)(struct sd *sd, struct mp_osd_res dim, int format,
|
|
double pts, struct sub_bitmaps *res);
|
|
char *(*get_text)(struct sd *sd, double pts);
|
|
};
|
|
|
|
struct lavc_conv;
|
|
struct lavc_conv *lavc_conv_create(struct mp_log *log, const char *codec_name,
|
|
char *extradata, int extradata_len);
|
|
char *lavc_conv_get_extradata(struct lavc_conv *priv);
|
|
char **lavc_conv_decode(struct lavc_conv *priv, struct demux_packet *packet);
|
|
void lavc_conv_reset(struct lavc_conv *priv);
|
|
void lavc_conv_uninit(struct lavc_conv *priv);
|
|
|
|
char *filter_SDH(struct sd *sd, char *format, int n_ignored, char *data, int length);
|
|
|
|
#endif
|