mirror of
https://github.com/mpv-player/mpv.git
synced 2024-09-20 03:52:22 +02:00
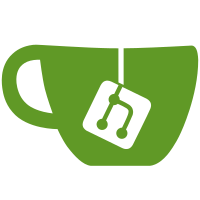
And also change input.conf to make all screenshots async. (Except the every-frame mode, which always uses synchronous mode and ignores the flag.) By default, the "screenshot" command is still asynchronous, because scripts etc. might depend on this behavior. This is only partially async. The code for determining the filename is still always run synchronously. Only encoding the screenshot and writing it to disk is asynchronous. We explicitly document the exact behavior as undefined, so it can be changed any time. Some of this is a bit messy, because I wanted to avoid duplicating the message display code between sync and async mode. In async mode, this is called from a worker thread, which is not safe because showing a message accesses the thread-unsafe OSD code. So the core has to be locked during this, which implies accessing the core and all that. So the code has weird locking calls, and we need to do core destruction in a more "controlled" manner (thus the outstanding_async field). (What I'd really want would be the OSD simply showing log messages instead.) This is pretty untested, so expect bugs. Fixes #4250.
49 lines
1.8 KiB
C
49 lines
1.8 KiB
C
/*
|
|
* This file is part of mpv.
|
|
*
|
|
* mpv is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2.1 of the License, or (at your option) any later version.
|
|
*
|
|
* mpv is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with mpv. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#ifndef MPLAYER_SCREENSHOT_H
|
|
#define MPLAYER_SCREENSHOT_H
|
|
|
|
#include <stdbool.h>
|
|
|
|
struct MPContext;
|
|
|
|
// One time initialization at program start.
|
|
void screenshot_init(struct MPContext *mpctx);
|
|
|
|
// Request a taking & saving a screenshot of the currently displayed frame.
|
|
// mode: 0: -, 1: save the actual output window contents, 2: with subtitles.
|
|
// each_frame: If set, this toggles per-frame screenshots, exactly like the
|
|
// screenshot slave command (MP_CMD_SCREENSHOT).
|
|
// osd: show status on OSD
|
|
void screenshot_request(struct MPContext *mpctx, int mode, bool each_frame,
|
|
bool osd, bool async);
|
|
|
|
// filename: where to store the screenshot; doesn't try to find an alternate
|
|
// name if the file already exists
|
|
// mode, osd: same as in screenshot_request()
|
|
void screenshot_to_file(struct MPContext *mpctx, const char *filename, int mode,
|
|
bool osd, bool async);
|
|
|
|
// mode is the same as in screenshot_request()
|
|
struct mp_image *screenshot_get_rgb(struct MPContext *mpctx, int mode);
|
|
|
|
// Called by the playback core code when a new frame is displayed.
|
|
void screenshot_flip(struct MPContext *mpctx);
|
|
|
|
#endif /* MPLAYER_SCREENSHOT_H */
|