mirror of
https://github.com/mpv-player/mpv.git
synced 2024-09-20 03:52:22 +02:00
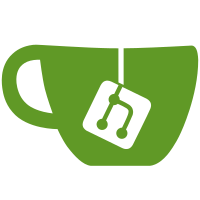
4e5d996c3a
added this as part of a series
of patches written to avoid wasteful sub redraws when playing a still
image with subs. The is_animated special case was specifically for ASS
subtitles that have animations/effects and would need repeated redraws
in the still image case. This check was done unconditionally for all ASS
subtitles, but for very big ASS subtitles, this text parsing can get a
bit expensive.
Because this function call is only ever needed for the weird edge case
of ASS subtitles over a still image, some additional logic can be added
to avoid calling is_animated in the vast majority of cases. The animated
field in demux_packet can be changed to a tristate instead where -1
indicates "unknown" (the default state). In update_subtitle, we can look
at the current state of the video tracks and decide whether or not it is
neccesary to perform is_animated and pass that knowledge to sd_ass
before any subtitle packets are decoded and thus save us from doing this
potentially expensive call.
65 lines
1.8 KiB
C
65 lines
1.8 KiB
C
#ifndef MPLAYER_DEC_SUB_H
|
|
#define MPLAYER_DEC_SUB_H
|
|
|
|
#include <stdbool.h>
|
|
#include <stdint.h>
|
|
|
|
#include "player/core.h"
|
|
#include "osd.h"
|
|
|
|
struct sh_stream;
|
|
struct mpv_global;
|
|
struct demux_packet;
|
|
struct mp_recorder_sink;
|
|
struct dec_sub;
|
|
struct sd;
|
|
|
|
enum sd_ctrl {
|
|
SD_CTRL_SUB_STEP,
|
|
SD_CTRL_SET_ANIMATED_CHECK,
|
|
SD_CTRL_SET_VIDEO_PARAMS,
|
|
SD_CTRL_SET_VIDEO_DEF_FPS,
|
|
SD_CTRL_UPDATE_OPTS,
|
|
};
|
|
|
|
enum sd_text_type {
|
|
SD_TEXT_TYPE_PLAIN,
|
|
SD_TEXT_TYPE_ASS,
|
|
SD_TEXT_TYPE_ASS_FULL,
|
|
};
|
|
|
|
struct sd_times {
|
|
double start;
|
|
double end;
|
|
};
|
|
|
|
struct attachment_list {
|
|
struct demux_attachment *entries;
|
|
int num_entries;
|
|
};
|
|
|
|
struct dec_sub *sub_create(struct mpv_global *global, struct track *track,
|
|
struct attachment_list *attachments, int order);
|
|
void sub_destroy(struct dec_sub *sub);
|
|
|
|
bool sub_can_preload(struct dec_sub *sub);
|
|
void sub_preload(struct dec_sub *sub);
|
|
void sub_redecode_cached_packets(struct dec_sub *sub);
|
|
void sub_read_packets(struct dec_sub *sub, double video_pts, bool force,
|
|
bool *packets_read, bool *sub_updated);
|
|
struct sub_bitmaps *sub_get_bitmaps(struct dec_sub *sub, struct mp_osd_res dim,
|
|
int format, double pts);
|
|
char *sub_get_text(struct dec_sub *sub, double pts, enum sd_text_type type);
|
|
char *sub_ass_get_extradata(struct dec_sub *sub);
|
|
struct sd_times sub_get_times(struct dec_sub *sub, double pts);
|
|
void sub_reset(struct dec_sub *sub);
|
|
void sub_select(struct dec_sub *sub, bool selected);
|
|
void sub_set_recorder_sink(struct dec_sub *sub, struct mp_recorder_sink *sink);
|
|
void sub_set_play_dir(struct dec_sub *sub, int dir);
|
|
bool sub_is_primary_visible(struct dec_sub *sub);
|
|
bool sub_is_secondary_visible(struct dec_sub *sub);
|
|
|
|
int sub_control(struct dec_sub *sub, enum sd_ctrl cmd, void *arg);
|
|
|
|
#endif
|