mirror of
https://github.com/mpv-player/mpv.git
synced 2024-09-20 03:52:22 +02:00
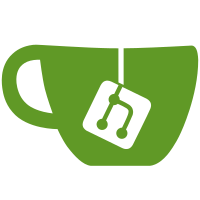
This affects non-ASS text subtitles (those which go through libavcodec's subtitle converter), which are muxed with video/audio. (Typically srt subs in mkv.) The problem is that seeking in the file can send a subtitle packet to the decoder multiple times. These packets are interlaved with video, and thus can't be all read when opening the file. Rather, subtitle packets can essentially be randomly skipped or repeated (by seeking). Until recently, this was solved by scanning the libass event list for duplicates. Then our builtin srt-to-ass converter was removed, and the problem was handled by fully clearing the subtitle list on each seek. This resulted in sub-seek not working properly for this type of file. Since the subtitle list was cleared on seek, it was not possible to do e.g. sub-seeks to subtitles before the current playback position. Fix this by not clearing the list, and intead explicitly rejecting duplicate packets. We use the packet file position was unique ID for subtitles; this is confirmed working for most file formats (although it is slightly risky - new demuxers may not necessarily set the file position to something unique, or at all). The list of seen packets is sorted, and the lookup uses binary search. This is to avoid quadratic complexity when subtitles are added in bulks, such as when opening a text subtitle file. In some places, the code has to be adjusted to pass through the packet file position correctly.
81 lines
2.2 KiB
C
81 lines
2.2 KiB
C
#ifndef MPLAYER_SD_H
|
|
#define MPLAYER_SD_H
|
|
|
|
#include "dec_sub.h"
|
|
#include "demux/packet.h"
|
|
|
|
// up to 210 ms overlaps or gaps are removed
|
|
#define SUB_GAP_THRESHOLD 0.210
|
|
// don't change timings if durations are smaller
|
|
#define SUB_GAP_KEEP 0.4
|
|
|
|
struct sd {
|
|
struct mp_log *log;
|
|
struct MPOpts *opts;
|
|
|
|
const struct sd_functions *driver;
|
|
void *priv;
|
|
|
|
const char *codec;
|
|
|
|
// Extra header data passed from demuxer
|
|
char *extradata;
|
|
int extradata_len;
|
|
|
|
struct sh_stream *sh;
|
|
|
|
// Set to !=NULL if the input packets are being converted from another
|
|
// format.
|
|
const char *converted_from;
|
|
|
|
// Video resolution used for subtitle decoding. Doesn't necessarily match
|
|
// the resolution of the VO, nor does it have to be the OSD resolution.
|
|
int sub_video_w, sub_video_h;
|
|
|
|
double video_fps;
|
|
|
|
// Shared renderer for ASS - done to avoid reloading embedded fonts.
|
|
struct ass_library *ass_library;
|
|
struct ass_renderer *ass_renderer;
|
|
pthread_mutex_t *ass_lock;
|
|
|
|
// Set by sub converter
|
|
const char *output_codec;
|
|
char *output_extradata;
|
|
int output_extradata_len;
|
|
|
|
// Internal buffer for sd_conv_* functions
|
|
struct sd_conv_buffer *sd_conv_buffer;
|
|
};
|
|
|
|
struct sd_functions {
|
|
const char *name;
|
|
bool accept_packets_in_advance;
|
|
bool (*supports_format)(const char *format);
|
|
int (*init)(struct sd *sd);
|
|
void (*decode)(struct sd *sd, struct demux_packet *packet);
|
|
void (*reset)(struct sd *sd);
|
|
void (*uninit)(struct sd *sd);
|
|
|
|
bool (*accepts_packet)(struct sd *sd); // implicit default if NULL: true
|
|
int (*control)(struct sd *sd, enum sd_ctrl cmd, void *arg);
|
|
|
|
// decoder
|
|
void (*get_bitmaps)(struct sd *sd, struct mp_osd_res dim, double pts,
|
|
struct sub_bitmaps *res);
|
|
char *(*get_text)(struct sd *sd, double pts);
|
|
|
|
// converter
|
|
struct demux_packet *(*get_converted)(struct sd *sd);
|
|
};
|
|
|
|
void sd_conv_add_packet(struct sd *sd, void *data, int data_len, double pts,
|
|
double duration, int64_t pos);
|
|
struct demux_packet *sd_conv_def_get_converted(struct sd *sd);
|
|
void sd_conv_def_reset(struct sd *sd);
|
|
void sd_conv_def_uninit(struct sd *sd);
|
|
|
|
#define SD_MAX_LINE_LEN 1000
|
|
|
|
#endif
|