mirror of
https://github.com/mpv-player/mpv.git
synced 2024-09-20 20:03:10 +02:00
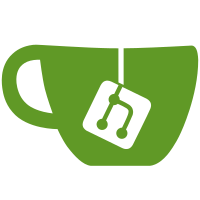
Back in the olden days, mpv's wayland backend was driven by the frame callback. This had several issues and was removed in favor of the current approach which allowed some advanced features (like display-resample and presentation time) to actually work properly. However as a consequence, it meant that mpv always rendered, even if the surface was hidden. Wayland people consider this "wasteful" (and well they aren't wrong). This commit aims to avoid wasteful rendering by doing some additional checks in the swapchain. There's three main parts to this. 1. Wayland EGL now uses an external swapchain (like the drm context). Before we start a new frame, we check to see if we are waiting on a callback from the compositor. If there is no wait, then go ahead and proceed to render the frame, swap buffers, and then initiate vo_wayland_wait_frame to poll (with a timeout) for the next potential callback. If we are still waiting on callback from the compositor when starting a new frame, then we simple skip rendering it entirely until the surface comes back into view. 2. Wayland on vulkan has essentially the same approach although the details are a little different. The ra_vk_ctx does not have support for an external swapchain and although such a mechanism could theoretically be added, it doesn't make much sense with libplacebo. Instead, start_frame was added as a param and used to check for callback. 3. For wlshm, it's simply a matter of adding frame callback to it, leveraging vo_wayland_wait_frame, and using the frame callback value to whether or not to draw the image.
28 lines
964 B
C
28 lines
964 B
C
#pragma once
|
|
|
|
#include "video/out/gpu/context.h"
|
|
#include "common.h"
|
|
|
|
struct ra_vk_ctx_params {
|
|
// See ra_swapchain_fns.get_vsync.
|
|
void (*get_vsync)(struct ra_ctx *ctx, struct vo_vsync_info *info);
|
|
|
|
// In case something special needs to be done when starting a frame.
|
|
bool (*start_frame)(struct ra_ctx *ctx);
|
|
|
|
// In case something special needs to be done on the buffer swap.
|
|
void (*swap_buffers)(struct ra_ctx *ctx);
|
|
};
|
|
|
|
// Helpers for ra_ctx based on ra_vk. These initialize ctx->ra and ctx->swchain.
|
|
void ra_vk_ctx_uninit(struct ra_ctx *ctx);
|
|
bool ra_vk_ctx_init(struct ra_ctx *ctx, struct mpvk_ctx *vk,
|
|
struct ra_vk_ctx_params params,
|
|
VkPresentModeKHR preferred_mode);
|
|
|
|
// Handles a resize request, and updates ctx->vo->dwidth/dheight
|
|
bool ra_vk_ctx_resize(struct ra_ctx *ctx, int width, int height);
|
|
|
|
// May be called on a ra_ctx of any type.
|
|
struct mpvk_ctx *ra_vk_ctx_get(struct ra_ctx *ctx);
|