mirror of
https://github.com/mpv-player/mpv.git
synced 2024-09-20 20:03:10 +02:00
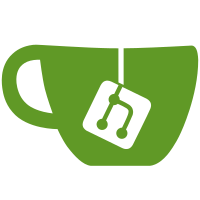
This has been a long standing annoyance - ffmpeg is removing sizeof(AVPacket) from the API which means you cannot stack-allocate AVPacket anymore. However, that is something we take advantage of because we use short-lived AVPackets to bridge from native mpv packets in our main decoding paths. We don't think that switching these to `av_packet_alloc` is desirable, given the cost of heap allocation, so this change takes a different approach - allocating a single packet in the relevant context and reusing it over and over. That's fairly straight-forward, with the main caveat being that re-initialising the packet is unintuitive. There is no function that does exactly what we need (what `av_init_packet` did). The closest is `av_packet_unref`, which additionally frees buffers and side-data. However, we don't copy those things - we just assign them in from our own packet, so we have to explicitly clear the pointers before calling `av_packet_unref`. But at least we can make a wrapper function for that. The weirdest part of the change is the handling of the vtt subtitle conversion. This requires two packets, so I had to pre-allocate two in the context struct. That sounds excessive, but if allocating the primary packet is too expensive, then allocating the secondary one for vtt subtitles must also be too expensive. This change is not conditional as heap allocated AVPackets were available for years and years before the deprecation.
56 lines
2.2 KiB
C
56 lines
2.2 KiB
C
/*
|
|
* This file is part of mpv.
|
|
*
|
|
* mpv is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2.1 of the License, or (at your option) any later version.
|
|
*
|
|
* mpv is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with mpv. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#ifndef MP_AVCOMMON_H
|
|
#define MP_AVCOMMON_H
|
|
|
|
#include <inttypes.h>
|
|
#include <stdbool.h>
|
|
|
|
#include <libavutil/avutil.h>
|
|
#include <libavutil/rational.h>
|
|
#include <libavcodec/avcodec.h>
|
|
|
|
struct mp_decoder_list;
|
|
struct demux_packet;
|
|
struct mp_codec_params;
|
|
struct AVDictionary;
|
|
struct mp_log;
|
|
|
|
int mp_lavc_set_extradata(AVCodecContext *avctx, void *ptr, int size);
|
|
enum AVMediaType mp_to_av_stream_type(int type);
|
|
AVCodecParameters *mp_codec_params_to_av(struct mp_codec_params *c);
|
|
int mp_set_avctx_codec_headers(AVCodecContext *avctx, struct mp_codec_params *c);
|
|
AVRational mp_get_codec_timebase(struct mp_codec_params *c);
|
|
void mp_set_av_packet(AVPacket *dst, struct demux_packet *mpkt, AVRational *tb);
|
|
int64_t mp_pts_to_av(double mp_pts, AVRational *tb);
|
|
double mp_pts_from_av(int64_t av_pts, AVRational *tb);
|
|
void mp_set_avcodec_threads(struct mp_log *l, AVCodecContext *avctx, int threads);
|
|
void mp_add_lavc_decoders(struct mp_decoder_list *list, enum AVMediaType type);
|
|
void mp_add_lavc_encoders(struct mp_decoder_list *list);
|
|
char **mp_get_lavf_demuxers(void);
|
|
int mp_codec_to_av_codec_id(const char *codec);
|
|
const char *mp_codec_from_av_codec_id(int codec_id);
|
|
bool mp_codec_is_lossless(const char *codec);
|
|
void mp_set_avdict(struct AVDictionary **dict, char **kv);
|
|
void mp_avdict_print_unset(struct mp_log *log, int msgl, struct AVDictionary *d);
|
|
int mp_set_avopts(struct mp_log *log, void *avobj, char **kv);
|
|
int mp_set_avopts_pos(struct mp_log *log, void *avobj, void *posargs, char **kv);
|
|
void mp_free_av_packet(AVPacket **pkt);
|
|
|
|
#endif
|