mirror of
https://github.com/mpv-player/mpv.git
synced 2024-09-20 20:03:10 +02:00
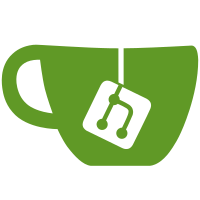
The memcpy_pic() function had a rather dangerous optimization: when the limit2width flag was not set, it was allowed to overwrite the data between the last pixel of a line and the first pixel of the next line (i.e. write over the stride padding). That was also the reason why there are so many whacky names for this function (memcpy_pic, my_memcpy_pic, memcpy_pic2). Kill this optimization, and never overwrite the stride padding. The code doing this can still be used if there's no stride padding at all, though. Also use the name memcpy_pic for the proper function. Now it should be rather clear that my_memcpy_pic and memcpy_pic2 are compatibility aliases. They should go away over the time.
74 lines
2.0 KiB
C
74 lines
2.0 KiB
C
/*
|
|
* This file is part of MPlayer.
|
|
*
|
|
* MPlayer is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2.1 of the License, or (at your option) any later version.
|
|
*
|
|
* MPlayer is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with MPlayer; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
|
|
*/
|
|
|
|
#ifndef MPLAYER_FASTMEMCPY_H
|
|
#define MPLAYER_FASTMEMCPY_H
|
|
|
|
#include "config.h"
|
|
#include <inttypes.h>
|
|
#include <string.h>
|
|
#include <stddef.h>
|
|
|
|
#define my_memcpy_pic memcpy_pic
|
|
#define memcpy_pic2(d, s, b, h, ds, ss, unused) memcpy_pic(d, s, b, h, ds, ss)
|
|
|
|
static inline void * memcpy_pic(void * dst, const void * src,
|
|
int bytesPerLine, int height,
|
|
int dstStride, int srcStride)
|
|
{
|
|
int i;
|
|
void *retval=dst;
|
|
|
|
if(bytesPerLine == dstStride && dstStride == srcStride)
|
|
{
|
|
if (srcStride < 0) {
|
|
src = (uint8_t*)src + (height-1)*srcStride;
|
|
dst = (uint8_t*)dst + (height-1)*dstStride;
|
|
srcStride = -srcStride;
|
|
}
|
|
|
|
memcpy(dst, src, srcStride*height);
|
|
}
|
|
else
|
|
{
|
|
for(i=0; i<height; i++)
|
|
{
|
|
memcpy(dst, src, bytesPerLine);
|
|
src = (uint8_t*)src + srcStride;
|
|
dst = (uint8_t*)dst + dstStride;
|
|
}
|
|
}
|
|
|
|
return retval;
|
|
}
|
|
|
|
static inline void memset_pic(void *dst, int fill, int bytesPerLine, int height,
|
|
int stride)
|
|
{
|
|
if (bytesPerLine == stride) {
|
|
memset(dst, fill, stride * height);
|
|
} else {
|
|
for (int i = 0; i < height; i++) {
|
|
memset(dst, fill, bytesPerLine);
|
|
dst = (uint8_t *)dst + stride;
|
|
}
|
|
}
|
|
}
|
|
|
|
#endif /* MPLAYER_FASTMEMCPY_H */
|