mirror of
https://github.com/mpv-player/mpv.git
synced 2024-09-20 20:03:10 +02:00
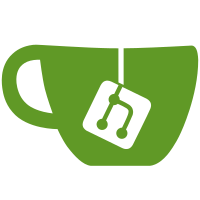
Stream implementations could set this to a unix file descriptor. The
generic stream code could use it as fallback for a few things. This
was confusing and insane. In most cases, the stream implementations
defined all callbacks, so setting the fd member didn't have any
advantages, other than avoiding defining a private struct to store it.
It appears that even if the stream implementation used close() on the
fd (or something equivalent), stream.c would close() it a second time
(and on windows, even would call closesocket()), which should be proof
for the insanity of this code.
For stream_file.c, additionally make sure we don't close stdin or
stdout if "-" is used as filename.
For stream_vcd.c, remove the control() code. This code most likely
didn't make the slightest sense, because it used a different type
for stream->priv. It also leaked memory. Maybe it worked, but it's
incorrect and insignificant anyway, so kill it. This code was added
with commit 9521c19
(svn commit 31019).
Untested for all protocols other than stream_file.c.
107 lines
2.0 KiB
C
107 lines
2.0 KiB
C
/* Imported from the dvbstream project
|
|
*
|
|
* Modified for use with MPlayer, for details see the changelog at
|
|
* http://svn.mplayerhq.hu/mplayer/trunk/
|
|
* $Id$
|
|
*/
|
|
|
|
#ifndef MPLAYER_DVBIN_H
|
|
#define MPLAYER_DVBIN_H
|
|
|
|
#include "config.h"
|
|
#include "stream.h"
|
|
|
|
#define SLOF (11700*1000UL)
|
|
#define LOF1 (9750*1000UL)
|
|
#define LOF2 (10600*1000UL)
|
|
|
|
#include <inttypes.h>
|
|
#include <linux/dvb/dmx.h>
|
|
#include <linux/dvb/frontend.h>
|
|
#include <linux/dvb/version.h>
|
|
|
|
#undef DVB_ATSC
|
|
#if defined(DVB_API_VERSION_MINOR)
|
|
|
|
/* kernel headers >=2.6.28 have version 5.
|
|
*
|
|
* FIXME: are there any real differences between 3.1 and 5?
|
|
*/
|
|
|
|
#if (DVB_API_VERSION == 3 && DVB_API_VERSION_MINOR >= 1) || DVB_API_VERSION == 5
|
|
#define DVB_ATSC 1
|
|
#endif
|
|
|
|
#endif
|
|
|
|
|
|
#define DVB_CHANNEL_LOWER -1
|
|
#define DVB_CHANNEL_HIGHER 1
|
|
|
|
#ifndef DMX_FILTER_SIZE
|
|
#define DMX_FILTER_SIZE 16
|
|
#endif
|
|
|
|
typedef struct {
|
|
char *name;
|
|
int freq, srate, diseqc, tone;
|
|
char pol;
|
|
int tpid, dpid1, dpid2, progid, ca, pids[DMX_FILTER_SIZE], pids_cnt;
|
|
fe_spectral_inversion_t inv;
|
|
fe_modulation_t mod;
|
|
fe_transmit_mode_t trans;
|
|
fe_bandwidth_t bw;
|
|
fe_guard_interval_t gi;
|
|
fe_code_rate_t cr, cr_lp;
|
|
fe_hierarchy_t hier;
|
|
} dvb_channel_t;
|
|
|
|
typedef struct {
|
|
uint16_t NUM_CHANNELS;
|
|
uint16_t current;
|
|
dvb_channel_t *channels;
|
|
} dvb_channels_list;
|
|
|
|
typedef struct {
|
|
int type;
|
|
dvb_channels_list *list;
|
|
char *name;
|
|
int devno;
|
|
} dvb_card_config_t;
|
|
|
|
typedef struct {
|
|
int count;
|
|
dvb_card_config_t *cards;
|
|
void *priv;
|
|
} dvb_config_t;
|
|
|
|
typedef struct {
|
|
int fd;
|
|
int card;
|
|
int fe_fd;
|
|
int sec_fd;
|
|
int demux_fd[3], demux_fds[DMX_FILTER_SIZE], demux_fds_cnt;
|
|
int dvr_fd;
|
|
|
|
dvb_config_t *config;
|
|
dvb_channels_list *list;
|
|
int tuner_type;
|
|
int is_on;
|
|
int retry;
|
|
int timeout;
|
|
int last_freq;
|
|
} dvb_priv_t;
|
|
|
|
|
|
#define TUNER_SAT 1
|
|
#define TUNER_TER 2
|
|
#define TUNER_CBL 3
|
|
#define TUNER_ATSC 4
|
|
|
|
int dvb_step_channel(stream_t *, int);
|
|
int dvb_set_channel(stream_t *, int, int);
|
|
dvb_config_t *dvb_get_config(void);
|
|
void dvb_free_config(dvb_config_t *config);
|
|
|
|
#endif /* MPLAYER_DVBIN_H */
|