mirror of
https://github.com/mpv-player/mpv.git
synced 2024-09-20 12:02:23 +02:00
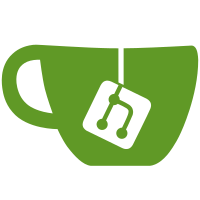
This fixes the "run" and "subprocess" commands on Windows, including youtube-dl support. Unix-like FD inheritance is emulated on Windows by using an undocumented data structure[1] that gets passed to the newly created process in STARTUPINFO.lpReserved2. It consists of two sparse arrays listing the HANDLE and internal CRT flags corresponding to each FD. This structure is used and understood primarily by MSVCRT, but there are other runtimes and frameworks that can write it, like libuv. The code for creating asynchronous "anonymous" pipes in Windows has been enhanced and moved into windows_utils.c. This is mainly an artifact of an unfinished future change to support anonymous IPC clients in Windows. Right now, it's still only used in subprocess-win.c [1]: https://www.catch22.net/tuts/undocumented-createprocess
50 lines
1.7 KiB
C
50 lines
1.7 KiB
C
/*
|
|
* This file is part of mpv.
|
|
*
|
|
* mpv is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2.1 of the License, or (at your option) any later version.
|
|
*
|
|
* mpv is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with mpv. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#ifndef MP_WINDOWS_UTILS_H_
|
|
#define MP_WINDOWS_UTILS_H_
|
|
|
|
#include <windows.h>
|
|
#include <stdbool.h>
|
|
|
|
// Conditionally release a COM interface and set the pointer to NULL
|
|
#define SAFE_RELEASE(u) \
|
|
do { if ((u) != NULL) (u)->lpVtbl->Release(u); (u) = NULL; } while(0)
|
|
|
|
char *mp_GUID_to_str_buf(char *buf, size_t buf_size, const GUID *guid);
|
|
#define mp_GUID_to_str(guid) mp_GUID_to_str_buf((char[40]){0}, 40, (guid))
|
|
char *mp_HRESULT_to_str_buf(char *buf, size_t buf_size, HRESULT hr);
|
|
#define mp_HRESULT_to_str(hr) mp_HRESULT_to_str_buf((char[256]){0}, 256, (hr))
|
|
#define mp_LastError_to_str() mp_HRESULT_to_str(HRESULT_FROM_WIN32(GetLastError()))
|
|
|
|
struct w32_create_anon_pipe_opts {
|
|
DWORD server_flags;
|
|
DWORD server_mode;
|
|
bool server_inheritable;
|
|
DWORD out_buf_size;
|
|
DWORD in_buf_size;
|
|
|
|
DWORD client_flags;
|
|
DWORD client_mode;
|
|
bool client_inheritable;
|
|
};
|
|
|
|
bool mp_w32_create_anon_pipe(HANDLE *server, HANDLE *client,
|
|
struct w32_create_anon_pipe_opts *opts);
|
|
|
|
#endif
|