mirror of
https://github.com/mpv-player/mpv.git
synced 2024-09-20 20:03:10 +02:00
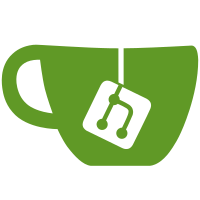
Wayland had some specific code that it used for implementing the presentation time protocol. It turns out that xorg's present extension is extremely similar, so it would be silly to duplicate this whole mess again. Factor this out to separate, independent code and introduce the mp_present struct which is used for handling the ust/msc values and some other associated values. Also, add in some helper functions so all the dirty details live specifically in present_sync. The only wayland-specific part is actually obtaining ust/msc values. Since only wayland or xorg are expected to use this, add a conditional to the build that only adds this file when either one of those are present. You may observe that sbc is completely omitted. This field existed in wayland, but was completely unused (presentation time doesn't return this). Xorg's present extension also doesn't use this so just get rid of it all together. The actual calculation is slightly altered so it is correct for our purposes. We want to get the presentation event of the last frame that was just occured (this function executes right after the buffer swap). The adjustment is to just remove the vsync_duration subtraction. Also, The overly-complicated queue approach is removed. This has no actual use in practice (on wayland or xorg). Presentation statistics are only ever used after the immediate preceding swap to update vsync timings or thrown away.
49 lines
1.6 KiB
C
49 lines
1.6 KiB
C
/*
|
|
* This file is part of mpv video player.
|
|
*
|
|
* mpv is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2.1 of the License, or (at your option) any later version.
|
|
*
|
|
* mpv is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with mpv. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#ifndef MP_PRESENT_SYNC_H
|
|
#define MP_PRESENT_SYNC_H
|
|
|
|
#include <stdbool.h>
|
|
#include <stdint.h>
|
|
#include "vo.h"
|
|
|
|
/* Generic helpers for obtaining presentation feedback from
|
|
* backend APIs. This requires ust/msc values. */
|
|
|
|
struct mp_present {
|
|
int64_t current_ust;
|
|
int64_t current_msc;
|
|
int64_t last_ust;
|
|
int64_t last_msc;
|
|
int64_t vsync_duration;
|
|
int64_t last_skipped_vsyncs;
|
|
int64_t last_queue_display_time;
|
|
};
|
|
|
|
// Used during the get_vsync call to deliver the presentation statistics to the VO.
|
|
void present_sync_get_info(struct mp_present *present, struct vo_vsync_info *info);
|
|
|
|
// Called after every buffer swap to update presentation statistics.
|
|
void present_sync_swap(struct mp_present *present);
|
|
|
|
// Called anytime the backend delivers new ust/msc values.
|
|
void present_update_sync_values(struct mp_present *present, int64_t ust,
|
|
int64_t msc);
|
|
|
|
#endif /* MP_PRESENT_SYNC_H */
|