mirror of
https://github.com/mpv-player/mpv.git
synced 2024-09-20 20:03:10 +02:00
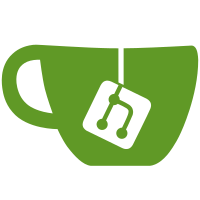
This only affects two special cases: printing subtitles to the terminal and printing subtitles on a still picture. Previously, mpv was very dumb here and spammed this logic on every single loop. For terminal subtitles, this isn't as big of a deal, but for the image case this is pretty bad. The entire VO constantly redrew even when there was no need to which can be very expensive depending on user settings. Instead, let's rework sub_read_packets so that it also tells us whether or not the subtitle packets update in some way in addition to telling us whether or not to read more. Since we cache all packets thanks to the previous commit, we can leverage this information to make a guess whether or not the current subtitle packet is supposed to be visible on the screen. Because the redraw now only happens when it is needed, the mp_set_timeout_hack can be removed.
63 lines
1.7 KiB
C
63 lines
1.7 KiB
C
#ifndef MPLAYER_DEC_SUB_H
|
|
#define MPLAYER_DEC_SUB_H
|
|
|
|
#include <stdbool.h>
|
|
#include <stdint.h>
|
|
|
|
#include "player/core.h"
|
|
#include "osd.h"
|
|
|
|
struct sh_stream;
|
|
struct mpv_global;
|
|
struct demux_packet;
|
|
struct mp_recorder_sink;
|
|
struct dec_sub;
|
|
struct sd;
|
|
|
|
enum sd_ctrl {
|
|
SD_CTRL_SUB_STEP,
|
|
SD_CTRL_SET_VIDEO_PARAMS,
|
|
SD_CTRL_SET_VIDEO_DEF_FPS,
|
|
SD_CTRL_UPDATE_OPTS,
|
|
};
|
|
|
|
enum sd_text_type {
|
|
SD_TEXT_TYPE_PLAIN,
|
|
SD_TEXT_TYPE_ASS,
|
|
};
|
|
|
|
struct sd_times {
|
|
double start;
|
|
double end;
|
|
};
|
|
|
|
struct attachment_list {
|
|
struct demux_attachment *entries;
|
|
int num_entries;
|
|
};
|
|
|
|
struct dec_sub *sub_create(struct mpv_global *global, struct track *track,
|
|
struct attachment_list *attachments, int order);
|
|
void sub_destroy(struct dec_sub *sub);
|
|
|
|
bool sub_can_preload(struct dec_sub *sub);
|
|
void sub_preload(struct dec_sub *sub);
|
|
void sub_redecode_cached_packets(struct dec_sub *sub);
|
|
void sub_read_packets(struct dec_sub *sub, double video_pts, bool force,
|
|
bool *packets_read, bool *sub_updated);
|
|
struct sub_bitmaps *sub_get_bitmaps(struct dec_sub *sub, struct mp_osd_res dim,
|
|
int format, double pts);
|
|
char *sub_get_text(struct dec_sub *sub, double pts, enum sd_text_type type);
|
|
char *sub_ass_get_extradata(struct dec_sub *sub);
|
|
struct sd_times sub_get_times(struct dec_sub *sub, double pts);
|
|
void sub_reset(struct dec_sub *sub);
|
|
void sub_select(struct dec_sub *sub, bool selected);
|
|
void sub_set_recorder_sink(struct dec_sub *sub, struct mp_recorder_sink *sink);
|
|
void sub_set_play_dir(struct dec_sub *sub, int dir);
|
|
bool sub_is_primary_visible(struct dec_sub *sub);
|
|
bool sub_is_secondary_visible(struct dec_sub *sub);
|
|
|
|
int sub_control(struct dec_sub *sub, enum sd_ctrl cmd, void *arg);
|
|
|
|
#endif
|