mirror of
https://github.com/obsproject/obs-studio.git
synced 2024-09-20 13:08:50 +02:00
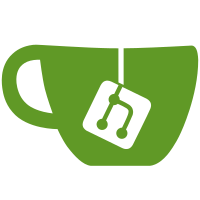
- Signals and dynamic callbacks now require declarations to be made before being used. What this does is allows us to get information about the functions dynamically which can be relayed to the user and plugins for future extended usage (this should have big implications later for scripting in particular, hopefully). - Reduced the number of types calldata uses from "everything I could think of" to simply integer, float, bool, pointer/object, string. Integer data is now stored as long long. Floats are now stored as doubles (check em). - Use a more consistent naming scheme for lexer error/warning macros. - Fixed a rather nasty bug where switching to an existing scene would cause it to increment sourceSceneRefs, which would mean that it would never end up never properly removing the source when the user clicks removed (stayed in limbo, obs_source_remove never got called)
62 lines
1.6 KiB
C
62 lines
1.6 KiB
C
/*
|
|
* Copyright (c) 2014 Hugh Bailey <obs.jim@gmail.com>
|
|
*
|
|
* Permission to use, copy, modify, and distribute this software for any
|
|
* purpose with or without fee is hereby granted, provided that the above
|
|
* copyright notice and this permission notice appear in all copies.
|
|
*
|
|
* THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
|
|
* WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
|
|
* MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
|
|
* ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
|
|
* WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
|
|
* ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF
|
|
* OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include "calldata.h"
|
|
#include "../util/darray.h"
|
|
|
|
#ifdef __cplusplus
|
|
extern "C" {
|
|
#endif
|
|
|
|
struct decl_param {
|
|
char *name;
|
|
enum call_param_type type;
|
|
uint32_t flags;
|
|
};
|
|
|
|
static inline void decl_param_free(struct decl_param *param)
|
|
{
|
|
if (param)
|
|
bfree(param->name);
|
|
memset(param, 0, sizeof(struct decl_param));
|
|
}
|
|
|
|
struct decl_info {
|
|
char *name;
|
|
const char *decl_string;
|
|
DARRAY(struct decl_param) params;
|
|
};
|
|
|
|
static inline void decl_info_free(struct decl_info *decl)
|
|
{
|
|
if (decl) {
|
|
for (size_t i = 0; i < decl->params.num; i++)
|
|
decl_param_free(decl->params.array+i);
|
|
da_free(decl->params);
|
|
|
|
bfree(decl->name);
|
|
memset(decl, 0, sizeof(struct decl_info));
|
|
}
|
|
}
|
|
|
|
EXPORT bool parse_decl_string(struct decl_info *decl, const char *decl_string);
|
|
|
|
#ifdef __cplusplus
|
|
}
|
|
#endif
|