mirror of
https://github.com/obsproject/obs-studio.git
synced 2024-09-20 04:42:18 +02:00
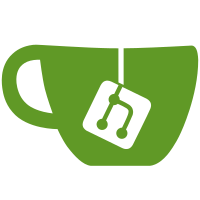
Introduce support for delivering BPM (Broadcast Performance Metrics) over SEI (for AVC/H.264 and HEVC/H.265) and OBU (for AV1) unregistered messages. Metrics being sent are the session frame counters, per-rendition frame counters, and RFC3339-based timestamping information to support end-to-end latency measurement. SEI/OBU messages are generated and sent with each IDR frame, and the frame counters are diff-based, meaning the counts reflect the diff between IDRs, not the running totals. BPM documentation is available at [1]. BPM relies on the recently introduced encoder packet timing support and the packet callback mechanism. BPM injection is enabled for an output by registering the `bpm_inject()` callback via `obs_output_add_packet_callback()` function. The callback must be unregistered using `obs_output_remove_packet_callback()` and `bpm_destroy()` must be used by the caller to release the BPM structures. It is important to measure the number of frames successfully encoded by the obs_encoder_t instances, particularly for renditions where the encoded frame rate differs from the canvas frame rate. The encoded_frames counter and `obs_encoder_get_encoded_frames()` API is introduced to measure and report this in the encoded rendition metrics message. [1] https://d50yg09cghihd.cloudfront.net/other/20240718-MultitrackVideoIntegrationGuide.pdf
52 lines
1.2 KiB
C
52 lines
1.2 KiB
C
// SPDX-FileCopyrightText: 2023 David Rosca <nowrep@gmail.com>
|
|
//
|
|
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
|
|
#pragma once
|
|
|
|
#include "util/c99defs.h"
|
|
|
|
#ifdef __cplusplus
|
|
extern "C" {
|
|
#endif
|
|
|
|
enum {
|
|
OBS_OBU_SEQUENCE_HEADER = 1,
|
|
OBS_OBU_TEMPORAL_DELIMITER = 2,
|
|
OBS_OBU_FRAME_HEADER = 3,
|
|
OBS_OBU_TILE_GROUP = 4,
|
|
OBS_OBU_METADATA = 5,
|
|
OBS_OBU_FRAME = 6,
|
|
OBS_OBU_REDUNDANT_FRAME_HEADER = 7,
|
|
OBS_OBU_TILE_LIST = 8,
|
|
OBS_OBU_PADDING = 15,
|
|
};
|
|
|
|
enum av1_obu_metadata_type {
|
|
METADATA_TYPE_HDR_CLL = 1,
|
|
METADATA_TYPE_HDR_MDCV,
|
|
METADATA_TYPE_SCALABILITY,
|
|
METADATA_TYPE_ITUT_T35,
|
|
METADATA_TYPE_TIMECODE,
|
|
METADATA_TYPE_USER_PRIVATE_6
|
|
};
|
|
|
|
/* Helpers for parsing AV1 OB units. */
|
|
|
|
EXPORT bool obs_av1_keyframe(const uint8_t *data, size_t size);
|
|
EXPORT void obs_extract_av1_headers(const uint8_t *packet, size_t size,
|
|
uint8_t **new_packet_data,
|
|
size_t *new_packet_size,
|
|
uint8_t **header_data, size_t *header_size);
|
|
|
|
EXPORT void metadata_obu_itu_t35(const uint8_t *itut_t35_buffer,
|
|
size_t itut_bufsize, uint8_t **out_buffer,
|
|
size_t *outbuf_size);
|
|
EXPORT void metadata_obu(const uint8_t *source_buffer, size_t source_bufsize,
|
|
uint8_t **out_buffer, size_t *outbuf_size,
|
|
uint8_t metadata_type);
|
|
|
|
#ifdef __cplusplus
|
|
}
|
|
#endif
|