mirror of
https://github.com/obsproject/obs-studio.git
synced 2024-09-20 13:08:50 +02:00
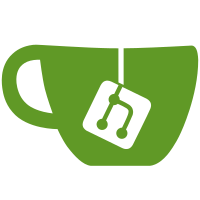
- Add a properties window for sources so that you can now actually edit the settings for sources. Also, display the source by itself in the window (Note: not working on mac, and possibly not working on linux). When changing the settings for a source, it will call obs_source_update on that source when you have modified any values automatically. - Add a properties 'widget', eventually I want to turn this in to a regular nice properties view like you'd see in the designer, but right now it just uses a form layout in a QScrollArea with regular controls to display the properties. It's clunky but works for the time being. - Make it so that swap chains and the main graphics subsystem will automatically use at least one backbuffer if none was specified - Fix bug where displays weren't added to the main display array - Make it so that you can get the properties of a source via the actual pointer of a source/encoder/output in addition to being able to look up properties via identifier. - When registering source types, check for required functions (wasn't doing it before). getheight/getwidth should not be optional if it's a video source as well. - Add an RAII OBSObj wrapper to obs.hpp for non-reference-counted libobs pointers - Add an RAII OBSSignal wrapper to obs.hpp for libobs signals to automatically disconnect them on destruction - Move the "scale and center" calculation in window-basic-main.cpp to its own function and in its own source file - Add an 'update' callback to WASAPI audio sources
128 lines
3.5 KiB
C++
128 lines
3.5 KiB
C++
/******************************************************************************
|
|
Copyright (C) 2014 by Hugh Bailey <obs.jim@gmail.com>
|
|
|
|
This program is free software: you can redistribute it and/or modify
|
|
it under the terms of the GNU General Public License as published by
|
|
the Free Software Foundation, either version 2 of the License, or
|
|
(at your option) any later version.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
GNU General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
******************************************************************************/
|
|
|
|
#include "obs-app.hpp"
|
|
#include "window-basic-properties.hpp"
|
|
#include "window-basic-main.hpp"
|
|
#include "qt-wrappers.hpp"
|
|
#include "display-helpers.hpp"
|
|
|
|
using namespace std;
|
|
|
|
OBSBasicProperties::OBSBasicProperties(QWidget *parent, OBSSource source_)
|
|
: QDialog (parent),
|
|
main (qobject_cast<OBSBasic*>(parent)),
|
|
resizeTimer (0),
|
|
ui (new Ui::OBSBasicProperties),
|
|
source (source_),
|
|
removedSignal (obs_source_signalhandler(source), "remove",
|
|
OBSBasicProperties::SourceRemoved, this)
|
|
{
|
|
setAttribute(Qt::WA_DeleteOnClose);
|
|
|
|
ui->setupUi(this);
|
|
|
|
OBSData settings = obs_source_getsettings(source);
|
|
obs_data_release(settings);
|
|
|
|
view = new OBSPropertiesView(settings,
|
|
obs_source_properties(source, App()->GetLocale()),
|
|
source, (PropertiesUpdateCallback)obs_source_update);
|
|
|
|
layout()->addWidget(view);
|
|
layout()->setAlignment(view, Qt::AlignRight);
|
|
view->show();
|
|
}
|
|
|
|
void OBSBasicProperties::SourceRemoved(void *data, calldata_t params)
|
|
{
|
|
QMetaObject::invokeMethod(static_cast<OBSBasicProperties*>(data),
|
|
"close");
|
|
|
|
UNUSED_PARAMETER(params);
|
|
}
|
|
|
|
void OBSBasicProperties::DrawPreview(void *data, uint32_t cx, uint32_t cy)
|
|
{
|
|
OBSBasicProperties *window = static_cast<OBSBasicProperties*>(data);
|
|
|
|
if (!window->source)
|
|
return;
|
|
|
|
uint32_t sourceCX = obs_source_getwidth(window->source);
|
|
uint32_t sourceCY = obs_source_getheight(window->source);
|
|
|
|
int x, y;
|
|
float scale;
|
|
|
|
GetScaleAndCenterPos(sourceCX, sourceCY, cx, cy, x, y, scale);
|
|
|
|
gs_matrix_push();
|
|
gs_matrix_scale3f(scale, scale, 1.0f);
|
|
gs_matrix_translate3f(-x, -y, 0.0f);
|
|
obs_source_video_render(window->source);
|
|
gs_matrix_pop();
|
|
}
|
|
|
|
void OBSBasicProperties::closeEvent(QCloseEvent *event)
|
|
{
|
|
main->UnloadProperties();
|
|
UNUSED_PARAMETER(event);
|
|
}
|
|
|
|
void OBSBasicProperties::resizeEvent(QResizeEvent *event)
|
|
{
|
|
if (isVisible()) {
|
|
if (resizeTimer)
|
|
killTimer(resizeTimer);
|
|
resizeTimer = startTimer(100);
|
|
}
|
|
|
|
UNUSED_PARAMETER(event);
|
|
}
|
|
|
|
void OBSBasicProperties::timerEvent(QTimerEvent *event)
|
|
{
|
|
if (event->timerId() == resizeTimer) {
|
|
killTimer(resizeTimer);
|
|
resizeTimer = 0;
|
|
|
|
QSize size = ui->preview->size();
|
|
obs_display_resize(display, size.width(), size.height());
|
|
}
|
|
}
|
|
|
|
void OBSBasicProperties::Init()
|
|
{
|
|
gs_init_data init_data = {};
|
|
|
|
show();
|
|
App()->processEvents();
|
|
|
|
QSize previewSize = ui->preview->size();
|
|
init_data.cx = uint32_t(previewSize.width());
|
|
init_data.cy = uint32_t(previewSize.height());
|
|
init_data.format = GS_RGBA;
|
|
QTToGSWindow(ui->preview->winId(), init_data.window);
|
|
|
|
display = obs_display_create(&init_data);
|
|
|
|
if (display)
|
|
obs_display_add_draw_callback(display,
|
|
OBSBasicProperties::DrawPreview, this);
|
|
}
|